Customizing Elevar's Data Layer
Learn how to transform existing events + build your own events.
If the event data our Data Layer sends by default doesn't work for you, you have two options:
- Option 1: Transform Existing Events
- Option 2: Build Your Own Events
Placement of your customization code will depend on what events or data you will be customizing. Events that occur before the checkout will be customized using a a snippet in your theme whereas customizations for events in the checkout and thank you page will be placed in the custom pixel, or if you haven't updated to the custom pixel yet your order status scripts. If your customization impacts your entire site, you'll want to place your customization code in both places.
Customizing events before your checkout
Elevar's code added via the App Theme Embed applies to your shoppers before they enter the checkout. You are unable to modify the App Theme Embed code, so we recommend that your create your own snippets that call our utilities. Although it is ultimately for you to decide how to go about doing this, here's the process we recommend for making such changes:
- Duplicate your theme into a theme with a name prefixed with "Elevar".
- Add a new snippet. Prefix the snippet's name with "elevar-" (for example,
elevar-customizations.liquid
):
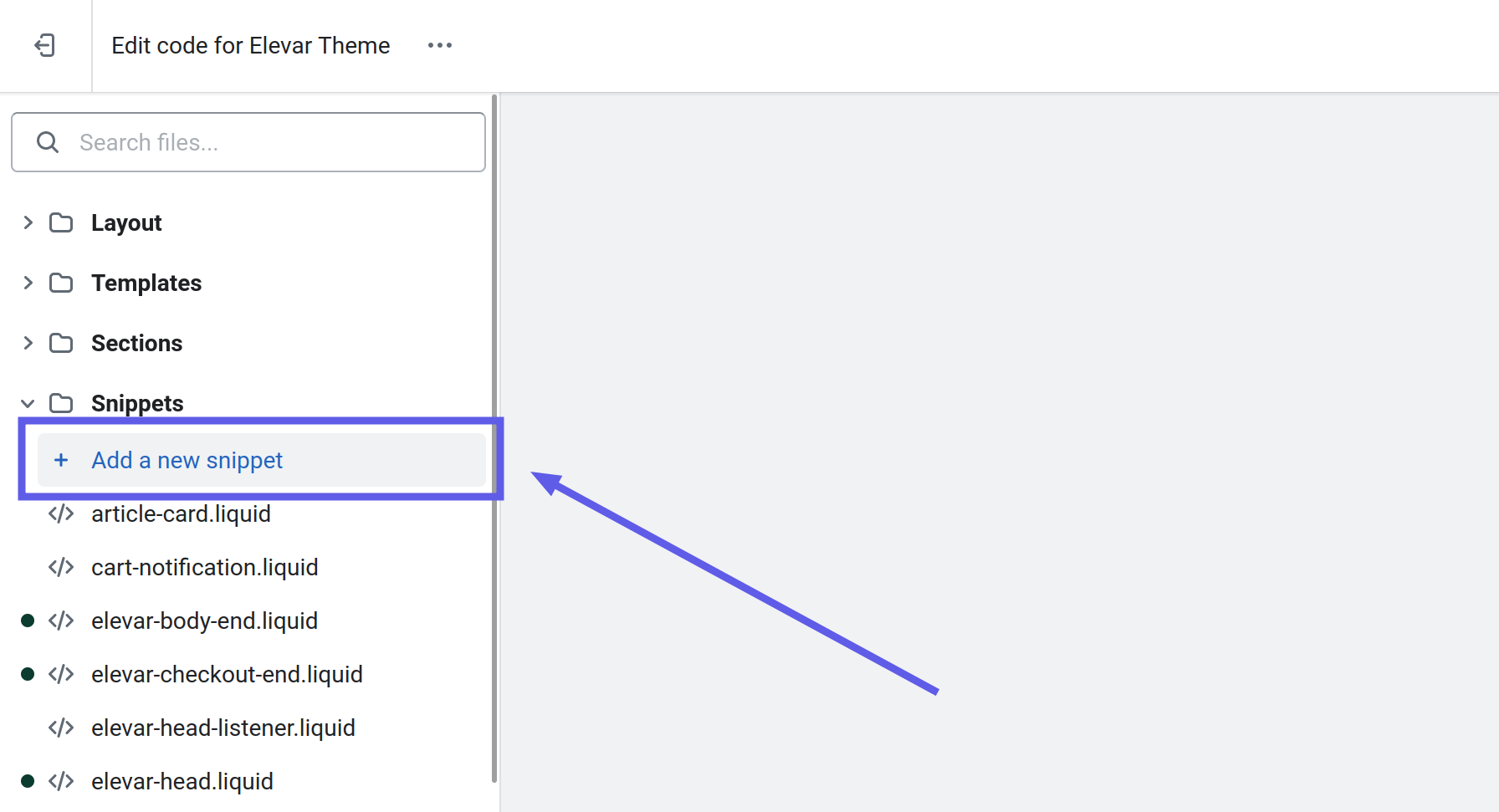
- Add the following to the snippet as a starting point:
<script>
// Add JavaScript code here - see options 1 + 2 in sections below
</script>
- Include the snippet in the
<head>
oftheme.liquid
and/orcheckout.liquid
(depending on if you want it to execute in checkout or not, and if you have access tocheckout.liquid
). Note that any custom snippets that are calling Elevar's utilities need to be placed below Elevar's snippets:
{% render 'elevar-customizations' %}
Next add in your code to transform existing events or build your own events.
Customizing checkout and thank you page events
As a part of your Elevar setup you manually added code to a Shopify Custom Pixel in the customer events. If you haven't upgraded to the custom pixel yet, your code for the thank you page lives in the order status page scripts in the checkout settings.
Custom Pixel
To access your custom pixel code going to the Settings in Shopify, and then navigate to the Customer events. You will need to place you customization code in the same Custom Pixel you've created for your checkout scripts. Your Elevar tracking script will not be able to reference if you place your customization code in a different custom pixel. You have likely named your Custom pixel "Elevar - Checkout Tracking" as shown below.
Add the following snippet as a starting point.
//Elevar Customizations - Do not remove when updating other Elevar script
// Add JavaScript code here - see options 1 + 2 in sections below
//End Elevar Customizations
Next add in your code to transform existing events or build your own events
Order Status Scripts
If you have not upgraded to the custom pixel you'll need to update your order status scripts. You'll access the order status page additional scripts by going to the Settings in Shopify, and then navigate to the Checkout settings, and then scroll to the Order status page additional scripts.
Add the following snippet as a starting point:
<!-- Elevar Customizations - Do not remove when updating other Elevar script -->
<script>
// Add JavaScript code here - see options 1 + 2 in sections below
</script>
<!-- End Elevar Customizations -->
Next add in your code to transform existing events or build your own events.
Option 1: Transform Existing Events
Before an event is pushed to our Data Layer, we look to see if window.ElevarTransformFn
exists. If it does exist, we call it, passing it the event that was going to be pushed. The return value of this function is what will now be pushed to the Data Layer. This allows you to write code to transform what data is sent in events.
For example, if you add the following code to your snippet:
window.ElevarTransformFn = item => {
if (item.event === "dl_add_to_cart") {
return {...item, custom_property: "my_custom_property"};
} else {
return item;
}
};
Any add to cart events with include the custom_property
value shown above.
console.log(window.dataLayer[...].custom_property); // logs `my_custom_property`
You are in control of the logic here, but there are few things to keep in mind:
- The function provided must always return a value. This means that if you are only wanting to transform certain events, make sure that you return the passed event object in all other cases.
- Do not directly mutate the event object that is passed into the function. This event object is shown in our debugger, so mutating it directly will result in a degraded debugging experience.
- We rely on all properties existing in the events we push, so to ensure that our app continues to work as expected, do not omit any properties that exist in the base event passed to the function.
- If you are wanting to modify an existing property's value (which we advise against in most cases, as it can easily break things), make sure that the value you use matches the existing value's type. For example, if the property contains a string, don't change it to contain a number.
Option 2: Build Your Own Events
There are two reasons why you'd want or need to build and send your own events via our Data Layer:
- The built-in event handlers don't work well for your sites theme (please let us know if this is the case, as we'll see if we can improve our built-in handlers to get them working on a wider selection of themes). If this is the case, be sure to turn off the relevant event in your Data Layer settings (in step 1) before continuing.
- You want to push your own client-side events to GTM but need to ensure that they include our contextual event information (user ID, device info, tracking cookie + query parameter values. etc.).
Both of these scenarios require you to use another one of our code utilities, window.ElevarDataLayer.push
.
For scenario 1, you'll need to ensure that the data pushed matches what our built-in handlers push:
window.ElevarDataLayer.push({ event: "dl_add_to_cart", ... });
For scenario 2, you can push whatever data you want (as it's up to you to read from it in GTM):
window.ElevarDataLayer.push({ event: "custom_event", ... });
Updated 2 days ago