Validating Data Layer Events
Follow this guide for the third step in the installation process for headless websites. Use the debugger in your Chrome Developer Console to QA your headless data layer install
Overview
This step must be completed on the headless site before deploying to production. The debugger can be utilized to validate and correct issues with the data layer events.
Enable or Disable the Debugger
- To enable the debugger, you can run the following in the console:
window?.ElevarInvalidateContext();
window.ElevarDebugMode(true);
- If you do not get any error, then this code will enable the debugger in your local storage and can be turned off by running the following in the console, or clearing your local storage.
window.ElevarDebugMode(false);
If you get any error like the following in your browser's console...
- Make sure that Elevar’s Non-Shopify Subdomain Script is placed as it is without any modifications on all the headless pages.
- If there are any modifications in the Non-Shopify Attribution Script, then it will throw this error on the page and the debugger will not work.
Example of Failed Data Layer Event:
- After enabling the debugger, you can start clicking through your site to test the data layer events. Here is an example of a failed data layer event:
window.ElevarDataLayer.push({
event: "dl_add_to_cart",
ecommerce: {
currencyCode: "USD",
add: {
actionField: {
list: "/collections/all"
},
products: [{
id: 41230516256821,
name: "Bedside Table",
brand: "Company 123",
category: "Indoor",
variant: null,
anotherField: true,
price: "69.99",
quantity: "1",
list: "/collections/all",
product_id: "6938075758645",
variant_id: "41230516256821",
image: "https://cdn.shopify.com/s/files/1/0588/1049/9125/products/dark-wall-bedside-table_925x_0a1b2f38-14b2-494f-a7ca-1ed1e79d924a.jpg?v=1656091291"
}]
}
}
});
- What is shown in the console is shown below.
- As we can see, the data layer object has incompatible fields for ecommerce.add.products[0].id and ecommerce.add.products[0].variant.
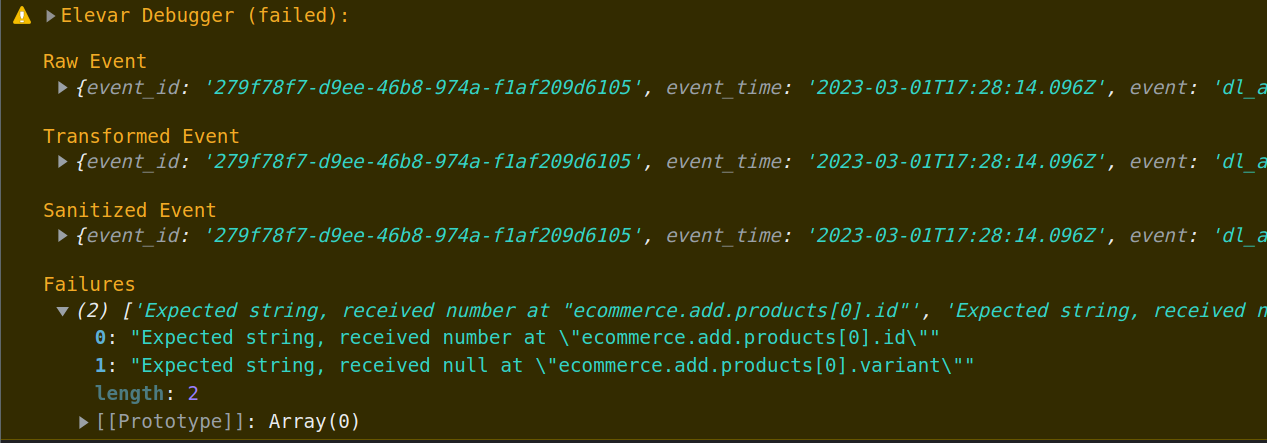
- We can correct this and run the data layer push again:
window.ElevarDataLayer.push({
event: “dl_add_to_cart”,
ecommerce: {
currencyCode: “USD”,
add: {
actionField: {
list: “/collections/all”
},
products: [{
id: “41230516256821",
name: “Bedside Table”,
brand: “Company 123",
category: “Indoor”,
variant: “12345324123423",
anotherField: true,
price: “69.99”,
quantity: “1",
list: “/collections/all”,
product_id: “6938075758645",
variant_id: “41230516256821”,
image: “https://cdn.shopify.com/s/files/1/0588/1049/9125/products/dark-wall-bedside-table_925x_0a1b2f38-14b2-494f-a7ca-1ed1e79d924a.jpg?v=1656091291”,
url: “https://domain.com/products/womens-shoe?variant=123”
}]
}
}
});
- And the event passes.

Updated 4 months ago
What’s Next